Windowsには標準でスケジュール管理機能が組み込まれていて、Outlookや標準のカレンダーアプリで表示させることができます。ここでは、自分のアプリからWindows標準のカレンダーにアクセスする方法を説明します。
開発環境
- .NET 6.0
- WinUI 3.0 (Windows App SDK 1.0)
- WinRT Build 22000
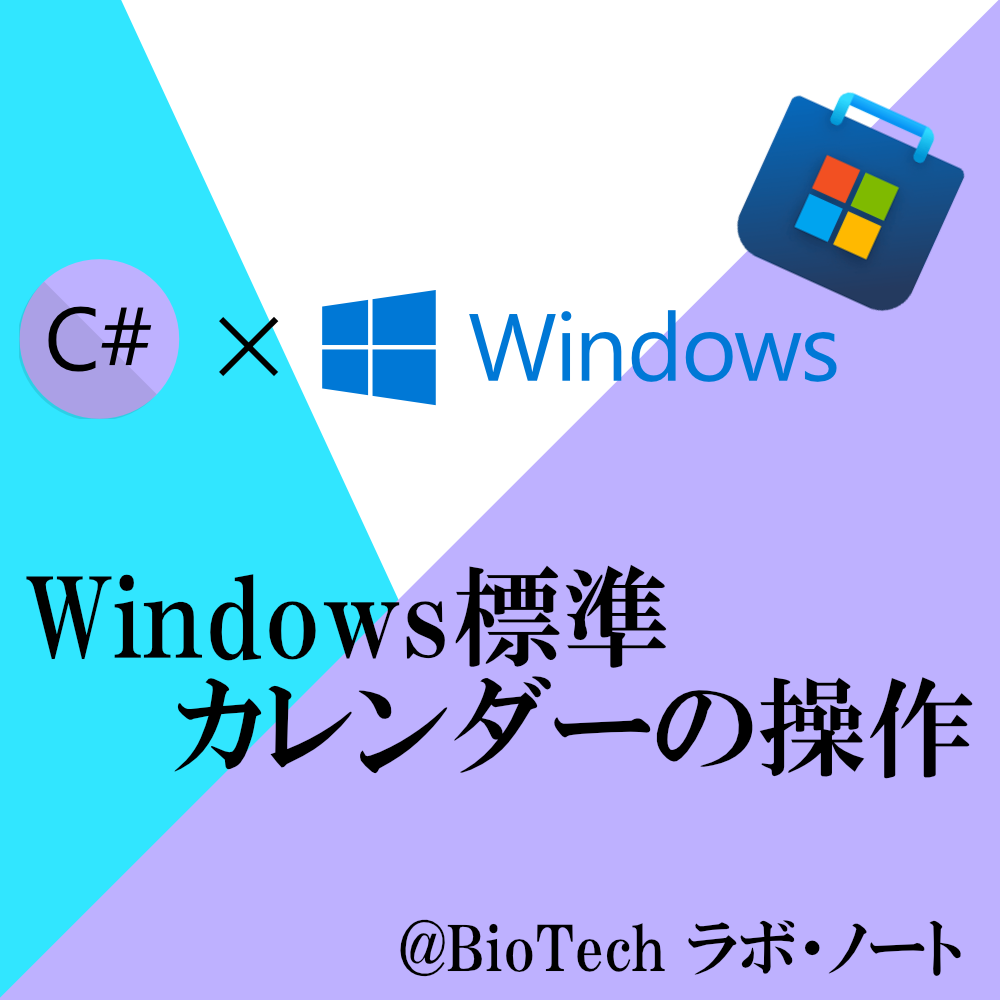
カレンダーとイベントを表すクラス
Windowsには標準でスケジュール管理機能が組み込まれていて、WinRT APIからそれを操作することも可能です。カレンダーとイベントを表すクラスは次のようになります。
- カレンダー → AppointmentCalendarクラス
- イベント → Appointmentクラス
また、Windowsの標準のカレンダーはAppointmentStoreという場所に保存されており、AppointmentStoreクラスからアクセス可能です。また、AppointmentStoreインスタンスはAppointmentManagerクラスのRequestStoreAsyncメソッドで取得できます。
Windows標準のカレンダーアプリのイベントもAPIから取得することが可能であり、またそのアプリ専用のカレンダーを作成することなども可能です。ただし、自分自身によって作成されたカレンダーには完全なアクセスが提供されますが、Outlookのカレンダーなど他のアプリによって作成されたカレンダーへのアクセスは制限されます。
ローカルのみでスケジュール管理を行う場合は、WinRT APIを用いてWindows標準のスケジュール管理機能を使うのがおススメですが、クラウド版のOutlookとの同期を行うような場合はMicrosoft Graphを用いて直接クラウド版のOutlookを操作した方が良いかもしれません。
カレンダーの操作
新しいカレンダーを作成する
AppointmentManagerクラスのRequestStoreAsyncメソッドで、まずカレンダーの保存場所であるAppointmentStoreを取得し、そのCreateAppointmentCalendarAsyncメソッドで新しいカレンダーを作成できます。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを作成する
await store.CreateAppointmentCalendarAsync("テストカレンダー");
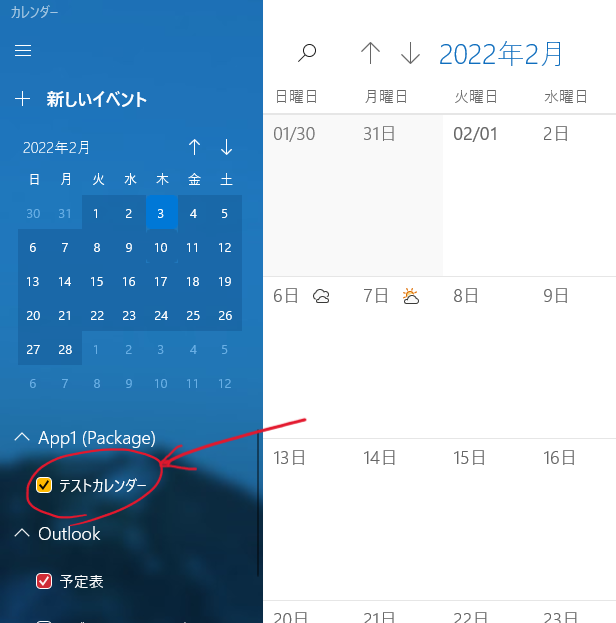
ただし、ここで作成したカレンダーはMicrosoftアカウントでWeb版のOutlookと同期するカレンダーとは別枠で作成されるので、クラウドとの同期はできずにローカルのカレンダーとなります。
既存のカレンダーを取得する
すべてのカレンダーの一覧を取得する
カレンダーの保存場所であるAppointmentStoreのFindAppointmentCalendarsAsyncメソッドで、AppointmentStoreに含まれるすべてのカレンダーを取得できます。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// すべてのカレンダーの一覧を取得する
var calendars = await store.FindAppointmentCalendarsAsync();
「テストカレンダー」という名前のカレンダーを取得する
指定した名前のカレンダーを取得する場合は、まずすべてのカレンダーを取得した上で、FirstOrDefaultメソッドで条件を指定して目的のカレンダーを抽出します。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを取得する
var calendars = await store.FindAppointmentCalendarsAsync();
AppointmentCalendar calendar = calendars.FirstOrDefault(x => x.DisplayName == "テストカレンダー");
ここで用いているLINQについては以下の記事をご覧ください。
特定のカレンダーを削除する
まずは削除したいカレンダーをAppointmentCalendarインスタンスとして取得し、そのDeleteAsyncメソッドでカレンダーを削除します。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを取得する
var calendars = await store.FindAppointmentCalendarsAsync();
AppointmentCalendar calendar = calendars.FirstOrDefault(x => x.DisplayName == "テストカレンダー");
// 取得したカレンダーを削除する
if (calendar != null)
{
await calendar.DeleteAsync();
}
なお、この操作では指定したカレンダーが警告なく削除されますので、注意して実行してください。
イベントの操作
イベントの操作を行うためには、まず上記の方法でそのイベントを登録するカレンダーのインスタンス(AppointmentCalendar)を取得します。
新しいイベントを作成する
イベントを表すAppointmentインスタンスを作成し、AppointmentCalendarのSaveAppointmentAsyncメソッドでそのイベントをカレンダーに登録します。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを取得する
var calendars = await store.FindAppointmentCalendarsAsync();
AppointmentCalendar calendar = calendars.FirstOrDefault(x => x.DisplayName == "テストカレンダー");
// イベントを作成する
Appointment appointment = new Appointment { Subject = "テストイベント"};
// イベントをカレンダーに登録する
if (calendar != null)
{
await calendar.SaveAppointmentAsync(appointment);
}
指定した期間のイベントを取得する
カレンダーを表すAppointmentCalendarインスタンスのFindAppointmentsAsyncメソッドで期間を指定することで、その期間のイベントのリストを取得できます。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを取得する
var calendars = await store.FindAppointmentCalendarsAsync();
AppointmentCalendar calendar = calendars.FirstOrDefault(x => x.DisplayName == "テストカレンダー");
// 現在から7日後までの間のイベントを取得する
var appointments = await calendar.FindAppointmentsAsync(DateTimeOffset.Now, new TimeSpan(7, 0, 0, 0));
既存のイベントを編集する
取得したイベント(Appointmentインスタンス)を編集したら、AppointmentCalendarのSaveAppointmentAsyncメソッドで再びカレンダーに登録することで保存できます。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを取得する
var calendars = await store.FindAppointmentCalendarsAsync();
AppointmentCalendar calendar = calendars.FirstOrDefault(x => x.DisplayName == "テストカレンダー");
// 現在から7日後までの間のイベントを取得する
var appointments = await calendar.FindAppointmentsAsync(DateTimeOffset.Now, new TimeSpan(7, 0, 0, 0));
// 取得したイベントに更新時間を記載する
foreach(Appointment appointment in appointments)
{
appointment.Details = "更新時間:" + DateTime.Now;
await calendar.SaveAppointmentAsync(appointment);
}
なお、この操作は他のアプリで作成したカレンダーに対してはアクセス権の関係で実行できません。
指定したイベントを削除する
削除するイベント(Appointmentインスタンス)のLocalIdを取得して、AppointmentCalendaのDeleteAppointmentAsyncメソッドの引数に指定することでそのイベントを削除できます。
AppointmentStore store = await AppointmentManager.RequestStoreAsync(AppointmentStoreAccessType.AllCalendarsReadWrite);
// 「テストカレンダー」というカレンダーを取得する
var calendars = await store.FindAppointmentCalendarsAsync();
AppointmentCalendar calendar = calendars.FirstOrDefault(x => x.DisplayName == "テストカレンダー");
// 現在から7日後までの間のイベントを取得する
var appointments = await calendar.FindAppointmentsAsync(DateTimeOffset.Now, new TimeSpan(7, 0, 0, 0));
// 取得したイベントを削除する
foreach(Appointment appointment in appointments)
{
string localId = appointment.LocalId;
await calendar.DeleteAppointmentAsync(localId);
}
なお、この操作は他のアプリで作成したカレンダーに対してはアクセス権の関係で実行できません。
コメント